Understanding Java: Counting Character Occurrences in Programs
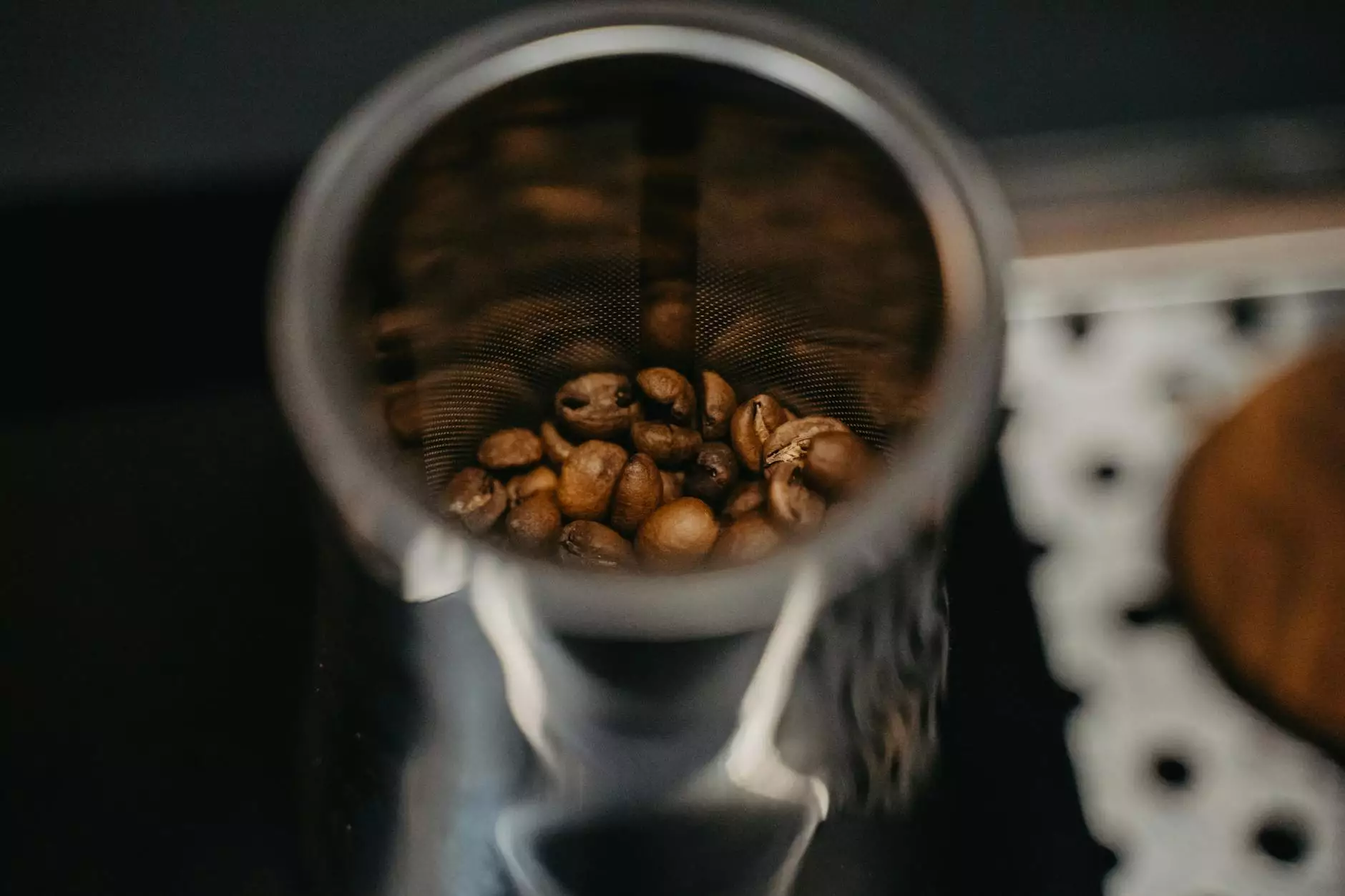
In the realm of programming, Java has solidified its position as one of the most widely used languages across various domains, including business applications, web development, and mobile apps. Among its myriad capabilities is the ability to manipulate and analyze data effectively. A pertinent example of Java’s functionality is encapsulated in the concept of counting character occurrences. This article discusses a practical implementation, specifically the Java program to count the occurrences of each character 0934, while also shedding light on its implications in data processing.
1. The Importance of Character Counting in Programming
Character counting can be critical for various applications, including:
- Data Analysis: Helps in understanding text data better by analyzing frequency and patterns.
- Data Validation: Ensures input data meets specified criteria and standards.
- Optimization: Supports optimizations in data storage by identifying redundant data.
- Text Processing: Assists in algorithms for search engines and natural language processing.
2. An Overview of the Java Language
Java is an object-oriented programming language known for its portability across platforms due to its “write once, run anywhere” capability. It emphasizes simplicity and accessibility, enabling developers to create complex applications efficiently. With its robust class libraries and frameworks, Java includes extensive support for tasks such as:
- Networking
- Database Connectivity
- Graphical User Interface (GUI) Development
- Multithreading
3. Exploring the Java Program to Count Character Occurrences
Now, let's delve into a basic implementation of a Java program that counts the occurrences of each character in a given string. This program can serve as a tool for multiple business applications, such as analyzing customer feedback or assessing text data in marketing strategies.
3.1. The Java Code
import java.util.HashMap; import java.util.Map; public class CharacterCounter { public static void main(String[] args) { String input = "swansondental.com"; countCharacterOccurrences(input); } public static void countCharacterOccurrences(String str) { Map characterCountMap = new HashMap(); for (char c : str.toCharArray()) { characterCountMap.put(c, characterCountMap.getOrDefault(c, 0) + 1); } System.out.println("Character occurrences in the string: "); for (Map.Entry entry : characterCountMap.entrySet()) { System.out.println(entry.getKey() + ": " + entry.getValue()); } } }3.2. Code Explanation
The provided Java code performs the following functions:
- Imports Necessary Libraries: The code utilizes HashMap and Map classes to store and count characters efficiently.
- Main Method: This method initializes a string (in this case, "swansondental.com") and calls the counting function.
- Character Counting Method: This method iterates over each character in the string, using a Map to tally occurrences.
- Output Results: After tallying the characters, the program outputs the result to the console.
4. Applications of Character Occurrence Counting in Business
Character occurrence counting can be leveraged in several business domains, including:
4.1. General Dentistry
In general dentistry, analyzing patient feedback through character counting can reveal insights into patient preferences and concerns. By addressing prevalent issues, practices can improve patient satisfaction and retention rates.
4.2. Cosmetic Dentistry
Cosmetic dentists can utilize character counting in scrutinizing marketing materials to ensure the right message reaches potential clients. By analyzing which terms occur most frequently, they can better tailor their advertising strategies.
4.3. Enhancing Customer Interaction
Regardless of the specific category, employing character counting helps businesses fine-tune their approaches, enhancing customer interaction and streamlining communication efforts.
5. Conclusion
The Java program to count the occurrences of each character 0934 illustrates how relatively simple programming constructs can be powerfully applied within various business contexts. By harnessing the capabilities of Java, businesses can achieve greater insights into their operations, ultimately leading to better decision-making and improved performance.
As programming continues to intertwine with business dynamics, mastering such fundamental techniques becomes indispensable for professionals looking to make a mark in their respective industries. Adapting these skills to real-world applications will undoubtedly foster growth and enhance competitive advantage.